Introduction
In today’s digital age, data security plays a crucial role in protecting sensitive information during transmission across networks. Encryption is a widely adopted technique to ensure data confidentiality. However, there are situations where the need arises to monitor and analyze encrypted network traffic, whether for security monitoring, troubleshooting, or debugging purposes. In this article, we will explore two key scenarios: one where we have access to the encryption key and another where we do not. We will accomplish this by using Python in conjunction with the Scapy library, offering insights into the world of network packet analysis and decryption.
Note: You can find the Server/Client program we use in this article on our website.
Scenario 1: Decryption with the Encryption Key
In the first scenario, we find ourselves in a fortunate position with access to the encryption key responsible for securing network traffic. Our objective is to intercept and decrypt these encrypted data packets in real-time as they traverse the network. Employing the versatile Scapy library, we will capture incoming network packets, and if they contain encrypted data, we will proceed to decrypt them using the provided encryption key.
Python Code for Scenario 1: Decryption with the Encryption Key
from scapy.all import *
from cryptography.fernet import Fernet
# Define the encryption key
key = b'your_secret_key_here' # Replace with your encryption key
def packet_handler(packet):
if packet.haslayer(IP):
src_ip = packet[IP].src
dst_ip = packet[IP].dst
print(f"IP Packet: Source IP={src_ip}, Destination IP={dst_ip}")
if packet.haslayer(TCP):
src_port = packet[TCP].sport
dst_port = packet[TCP].dport
print(f"TCP Packet: Source Port={src_port}, Destination Port={dst_port}")
if packet.haslayer(Raw):
raw_data = packet[Raw].load
try:
decrypted_data = Fernet(key).decrypt(raw_data).decode("utf-8")
print(f"Decrypted Data: {decrypted_data}")
except Exception as e:
print(f"Decryption error: {e}")
if packet.haslayer(UDP):
src_port = packet[UDP].sport
dst_port = packet[UDP].dport
print(f"UDP Packet: Source Port={src_port}, Destination Port={dst_port}")
print("-" * 50)
# Use sniff function to listen to network traffic
# Replace "lo" with the network interface you want to listen on
sniff(iface="lo", prn=packet_handler, filter="tcp or udp")
The Output
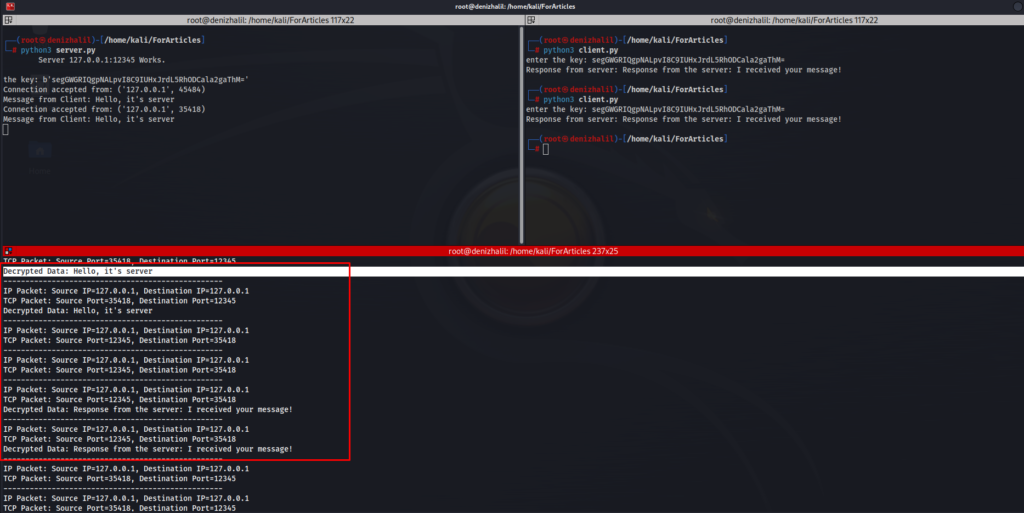
Scenario 2: Analyzing Encrypted Traffic without the Encryption Key
The second scenario presents a more challenging situation where we lack access to the encryption key. Nevertheless, we can leverage Python and Scapy to intercept network traffic, allowing us to gain insights into the metadata of the encrypted packets. While we cannot decrypt the content directly without the key, we can still extract valuable information, such as source and destination IP addresses, source and destination ports, and any unencrypted data contained within the packets.
DeepNot: Those who want to learn Python, do not forget to check out my Python in 30 days series that I prepared for you.
Python Code for Scenario 2: Analyzing Encrypted Traffic without the Encryption Key
from scapy.all import *
def packet_handler(packet):
if packet.haslayer(IP):
src_ip = packet[IP].src
dst_ip = packet[IP].dst
print(f"IP Packet: Source IP={src_ip}, Destination IP={dst_ip}")
if packet.haslayer(TCP):
src_port = packet[TCP].sport
dst_port = packet[TCP].dport
print(f"TCP Packet: Source Port={src_port}, Destination Port={dst_port}")
if packet.haslayer(Raw):
raw_data = packet[Raw].load
print(f"Raw Data: {raw_data}")
if packet.haslayer(UDP):
src_port = packet[UDP].sport
dst_port = packet[UDP].dport
print(f"UDP Packet: Source Port={src_port}, Destination Port={dst_port}")
print("-" * 50)
# Use sniff function to listen to network traffic
# Replace "lo" with the network interface you want to listen on
sniff(iface="lo", prn=packet_handler, filter="tcp or udp")
The Output
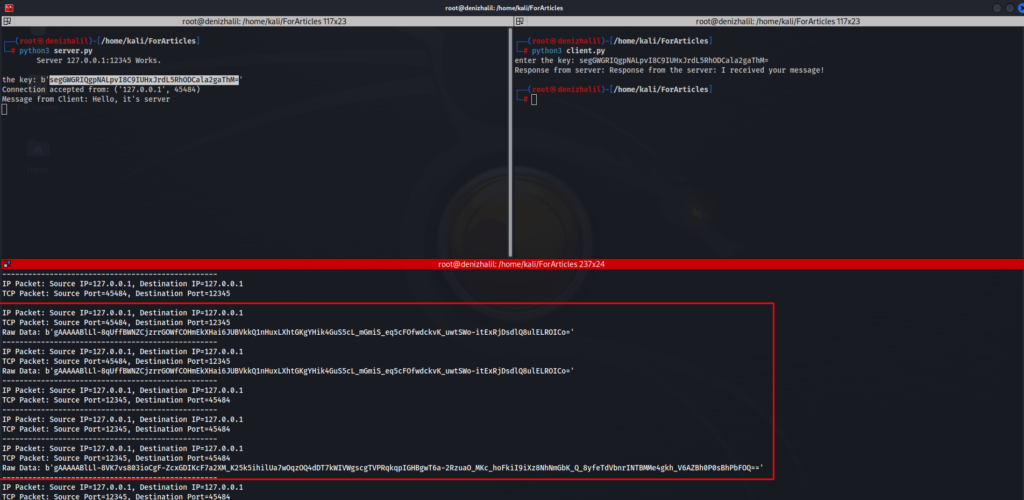
Conclusion
Decrypting encrypted network traffic can be a valuable skill for network administrators, security professionals, and developers. Understanding the scenarios in which we operate, whether we possess the necessary encryption keys, and the associated limitations is crucial when dealing with encrypted traffic. Python, along with the Scapy library, provides a robust toolkit for network packet analysis, offering insights into network communication and ensuring the security of our systems.
It’s important to emphasize that any interception and analysis of network traffic must be conducted ethically and in compliance with applicable laws and regulations.
In summary, this article has explored two scenarios for decrypting encrypted network traffic using Python and Scapy, highlighting the significance of encryption keys and the constraints when the key is unavailable.
Muhteşem olmuş
elinize sağlık teşekkür ederim