What is a Socket?
Welcome to our comprehensive guide on Python Socket Programming, an essential skill for network communication and security. This article delves into the intricacies of Python’s socket module, offering a detailed exploration of both TCP and UDP sockets, their uses, and how they differ. We start by introducing the concept of sockets in Python, a pivotal tool for network-based application development. Whether you’re a beginner or an experienced programmer, this guide provides valuable insights into creating socket clients and servers, managing network connections, and troubleshooting common issues in socket programming. By integrating practical examples and easy-to-follow code snippets, our aim is to enhance your understanding and skills in Python network programming, a vital component in today’s interconnected world.
Basic Socket Types:
- TCP Sockets: Used for reliable, ordered, and error-checked communication based on connections.
- UDP Sockets: Used for connectionless communication, faster but less reliable.
Sockets serve as a bridge between devices on a network, ensuring that data packets reach their correct destinations.
Working with Sockets in Python
Python simplifies socket programming with its socket
module. This module allows you to engage in data exchange using network sockets.
Creating a Simple Socket:
To create a TCP socket in Python, you can follow these steps:
- 1. Importing the Socket Module:
import socket
- 2. Creating a Socket Object:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Here, AF_INET
indicates the use of IPv4 and SOCK_STREAM
indicates the creation of a TCP-based socket.
- 3. Connecting to a Server: You can connect using the server’s IP address and port number. For example, to connect to a server running on the local machine:
s.connect(('localhost', 12345))
Here, 12345
is the port number the server is listening on.
This simple example demonstrates the basic steps of creating a TCP client socket in Python. More complex socket programs can involve sending and receiving data, creating servers, and asynchronous operations.
1. TCP vs UDP Sockets: Key Differences and Use Cases
TCP (Transmission Control Protocol) and UDP (User Datagram Protocol) are two fundamental protocols used for data transmission over a network. Both work alongside the Internet Protocol (IP), but offer different approaches to data transfer.
TCP Sockets
- Reliable Communication: TCP ensures data is delivered in the correct order and without errors. If a packet is lost, TCP resends it.
- Connection-Based: A connection is established between two devices before communication starts and is terminated at the end.
- Data Flow Control: TCP regulates network traffic, controlling the flow of data, preventing network congestion.
- Use Cases: Ideal for sending emails, loading web pages, file transfers, etc.
UDP Sockets
- Connectionless Communication: UDP sends data directly without establishing a connection, speeding up communication but compromising reliability.
- Fast Communication: Data packets are sent without error checking or ordering, enabling quicker communication.
- Limited Error Control: Lost packets are not resent. This can be problematic for some applications.
- Use Cases: Preferred for live video or audio streaming, online gaming, and certain IoT applications.
Comparison
- Reliability: TCP guarantees accurate and complete data delivery, while UDP does not offer such guarantees.
- Speed: UDP is faster than TCP as it incorporates fewer control mechanisms.
- Complexity: TCP is more complex, encompassing connection management and data flow control mechanisms.
Generally, TCP is chosen when data accuracy is more important than speed, and UDP is used when speed is more critical. Each protocol offers advantages and disadvantages depending on specific needs.
2. Finding an IP Address: Retrieving the IP Address of Any Website
This section will explain how to find the IP address of any website using Python. This process typically involves resolving a domain name to an IP address (DNS lookup).
Example of Finding an IP Address with Python
The socket
module, one of Python’s standard libraries, can be used for this task:
import socket
def get_ip_address(url):
ip_address = socket.gethostbyname(url)
return ip_address
# Example usage
url = "www.example.com"
print(f"The IP address of {url} is: {get_ip_address(url)}")
This simple function returns the IP address of the given URL. Here, the socket.gethostbyname()
function resolves the domain name to retrieve the corresponding IP address.
3. Port Scanning: Scanning for Open Ports on a Given Target
Port scanning is used to identify open ports on devices in a network. It is commonly used in security testing or network management.
Simple Port Scanning Example with Python
You can use the socket
module in Python for a simple port scanning operation. Here is an example of a basic port scanning script:
import socket
def scan_ports(host, port_range):
open_ports = []
for port in range(*port_range):
with socket.socket(socket.AF_INET, socket.SOCK_STREAM) as s:
s.settimeout(1)
if s.connect_ex((host, port)) == 0:
open_ports.append(port)
return open_ports
# Example usage
host = "192.168.1.1" # Example IP address
port_range = (20, 1024)
print(f"Open ports at {host}: {scan_ports(host, port_range)}")
This script scans for open ports at the specified IP address and port range. The socket.connect_ex()
method attempts to connect to a specific port and returns an error code if it fails. A successful connection indicates that the port is open and it is added to the list.
4. Creating a Socket Server: Step-by-Step Guide
In this section, we will explore how to create a simple socket server using Python, step by step. A socket server is a program that accepts client connections over a network and exchanges data with them.
Step 1: Creating a Socket
The first step in creating a socket server in Python is to create a socket object. This is done using the socket.socket()
function.
import socket
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Here, the AF_INET
parameter indicates the use of IPv4 addressing, and SOCK_STREAM
signifies the creation of a TCP-based socket.
Step 2: Binding the Socket to an Address
The created socket must be bound to an IP address and port number. This is achieved using the bind()
method.
host = '127.0.0.1' # Local server address
port = 12345 # A random port number
server_socket.bind((host, port))
Step 3: Starting to Listen on the Socket
The server begins listening for connection requests with the listen()
method.
server_socket.listen()
print(f"Server is listening on {host}:{port}.")
Step 4: Accepting Connections and Exchanging Data
The server accepts incoming connections using the accept()
method and can then exchange data over this connection.
client_socket, address = server_socket.accept()
print(f"Connection accepted from {address}.")
You can read incoming data and send responses.
message = client_socket.recv(1024).decode() # Reading incoming data
print(f"Incoming message: {message}")
response = "Hello, Client!"
client_socket.send(response.encode()) # Sending a response
client_socket.close() # Closing the connection
Step 5: Closing the Server
server_socket.close()
Example Server Script
Combining all these steps, we get a simple socket server script like the following:
import socket
def start_server():
host = '127.0.0.1'
port = 12345
server_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
server_socket.bind((host, port))
server_socket.listen()
print(f"Server is listening on {host}:{port}.")
client_socket, address = server_socket.accept()
print(f"Connection accepted from {address}.")
message = client_socket.recv(1024).decode()
print(f"Incoming message: {message}")
response = "Hello, Client!"
client_socket.send(response.encode())
client_socket.close()
server_socket.close()
if __name__ == "__main__":
start_server()
This script starts a basic TCP socket server, accepts a client connection, prints the incoming message, sends a response, and then closes the connection. This basic example demonstrates the core principles of socket programming and serves as a foundation for more complex applications.
5. Creating a Socket Client: Basic Steps
In this section, we’ll step-by-step go through how to create a simple socket client using Python. A socket client is a program that connects to a server and exchanges data with it.
Step 1: Creating a Socket
The first step in creating a socket client is to create a socket object using the socket.socket()
function.
import socket
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
Here AF_INET
indicates the use of IPv4 addressing, and SOCK_STREAM
means a TCP-based socket will be used.
Step 2: Connecting to the Server
The client connects to the server using the connect()
method with the server’s IP address and port number.
host = '127.0.0.1' # The server's IP address
port = 12345 # The port number the server is listening on
client_socket.connect((host, port))
Step 3: Sending and Receiving Data
Once connected, the client can send data to the server and receive data from the server.
message = "Hello, Server!"
client_socket.send(message.encode()) # Sending a message to the server
response = client_socket.recv(1024).decode() # Receiving a response from the server
print(f"Response from the server: {response}")
Step 4: Closing the Connection
After communication is complete, the client should close the connection. This is done with the close()
method.
client_socket.close()
Example Client Script
By combining the above steps, you can create a simple socket client script like this:
import socket
def start_client():
host = '127.0.0.1'
port = 12345
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((host, port))
message = "Hello, Server!"
client_socket.send(message.encode())
response = client_socket.recv(1024).decode()
print(f"Response from the server: {response}")
client_socket.close()
if __name__ == "__main__":
start_client()
This script connects to the server, sends a message, receives a response from the server, and then closes the connection. This basic example demonstrates the fundamental principles of socket clients and provides a foundation for more complex applications. Socket programming is an important tool, especially in network communication and distributed systems. With these basics, you can move on to more advanced socket programming projects.
6. Error Management in Socket Programming: Common Problems and Solutions
Error management in socket programming is crucial for developing stable and reliable applications. In this section, we’ll explore some common problems encountered in socket programming and how to address them.
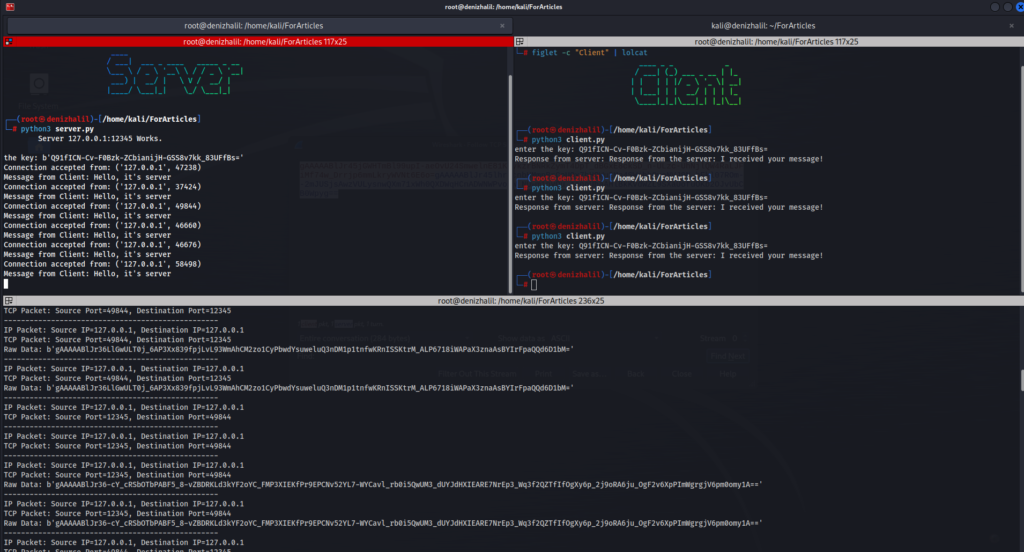
Connection Errors
- Problem: The server is unreachable or connection is refused.
- Solution: These errors often stem from incorrect IP address/port information or the server being down. Verify the IP address and port details, and check if the server is operational.
Timeout Errors
- Problem: Connections or responses time out.
- Solution: Use the
settimeout()
method to set a timeout duration for sockets. This prevents the program from hanging on connections that take too long to respond.
Data Transmission Errors
- Problem: Sent/Received data is different from expected or incomplete.
- Solution: Check the size of the data and ensure that all parts of the data are completely sent and received. While TCP has its own retry mechanisms, it’s beneficial to perform additional checks to ensure data integrity.
Socket Closure Errors
- Problem: Sockets are not closing properly.
- Solution: Ensure sockets are closed properly using the
close()
method after each use. Connections closed unexpectedly by the client or server can lead to wasted resources.
Managing Multiple Connections
- Problem: The server struggles to manage multiple connections simultaneously.
- Solution: Use threading or asynchronous programming techniques to manage multiple connections. This allows the server to communicate effectively with multiple clients at the same time.
Security-Related Issues
- Problem: Communication over sockets is not secure.
- Solution: Use encryption protocols like SSL/TLS for transmitting sensitive data. Also, consider using firewalls and authentication mechanisms to prevent unauthorized access.
Debugging Tips
- Detailed Logging: Log errors and important steps encountered during operations. This helps in identifying the source of problems.
- Custom Error Classes: Create customized error classes to better manage different types of errors.
- User Feedback: Provide understandable error messages to users on both client and server sides. This improves the user experience.
Error management in socket programming is a critical component in enhancing the robustness and reliability of your application. Properly addressing errors ensures your application is more resilient and user-friendly.
Conclusion
Socket programming is a fundamental pillar of modern network communication, and Python offers a robust set of tools in this area. The topics covered in this article range from the basic definition of sockets to creating socket servers and clients in Python, and advanced topics like error management. Understanding the basic concepts and practices enables the development of more complex network-based applications.
Socket programming is especially critical in fields like network security, data communication, and distributed systems. Understanding the differences between TCP and UDP helps in selecting the right tools for your application’s needs. Techniques like IP address retrieval and port scanning are fundamental skills in network security and management. Creating socket servers and clients forms the foundation of network-based applications, while error management ensures these applications are robust and reliable.
We hope this guide serves as a valuable resource for those looking to get started with Python socket programming. Grasping the fundamentals of socket programming can help you advance in this field and gain a deeper understanding of network communication. Remember, practice is an integral part of the learning process. Working on the provided examples and guides is the best way to enhance your skills. Socket programming is an evolving field, and becoming proficient in it will always be a valuable skill in the world of technology.
I don’t een know how I ended up here, but I thought this poxt was great.