Introduce
In the dynamic world of software development, ‘Encapsulation in Programming’ stands as a fundamental principle, especially in object-oriented programming (OOP). This article dives deep into encapsulation, a strategic approach that not only enhances code robustness and security but also clarifies its structure. Encapsulation involves bundling data and methods within a class and controlling access to this data, establishing a protective barrier against misuse and interference.
The Importance of Encapsulation
Encapsulation isn’t merely about keeping data and methods together; it’s fundamentally about defining the external interface that the rest of your application interacts with and protecting the internal workings of that interface. By doing so, it serves several crucial purposes: for clean code
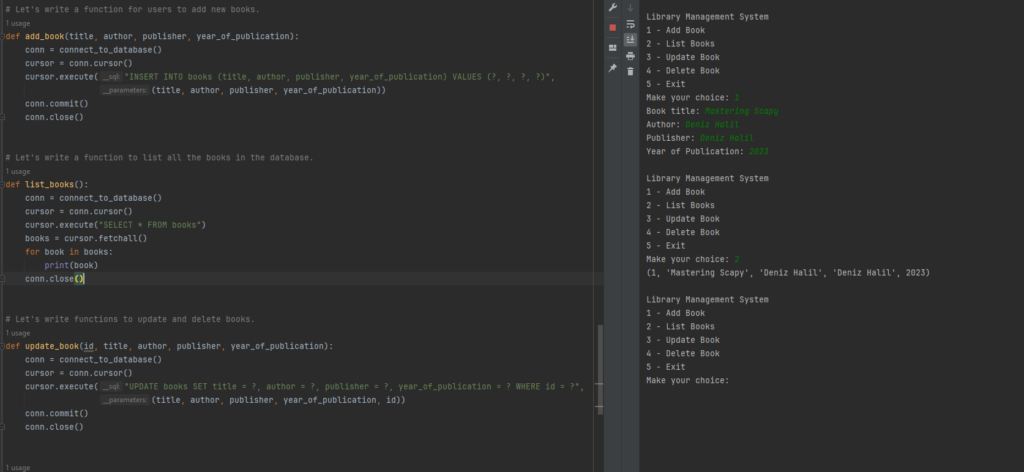
- Security: Encapsulation shields an object’s internal state from unauthorized access and modification. This means critical data remains safe from inadvertent changes that can cause bugs or security vulnerabilities.
- Simplicity and Modularity: By exposing only necessary components of a class, encapsulation simplifies the interaction with an object. This modularity makes the code more manageable and comprehensible.
- Flexibility and Maintainability: Changes to the internal workings of a class do not affect other parts of the program as long as the interface remains consistent. This encapsulation aspect facilitates easier maintenance and enhancement of the system over time.
- Reduction of Complexity and Increase in Clarity: Encapsulation helps in organizing the code better, making it more readable and easier to understand. This is especially beneficial in large codebases and team environments.
Basic Example: The Car Class
Let’s illustrate encapsulation with a basic Python example:
class Car:
def __init__(self, model, year):
self.__model = model # private attribute
self.__year = year # private attribute
def get_model(self):
return self.__model
def set_model(self, model):
self.__model = model
def get_year(self):
return self.__year
def set_year(self, year):
self.__year = year
# Create a Car object
my_car = Car("Toyota", 2020)
# Access and modify attributes via methods
print(my_car.get_model()) # Access model
my_car.set_model("Honda") # Modify model
print(my_car.get_model()) # Access modified model
In this example:
- The
Car
class encapsulates data (model
andyear
) and methods (get_model
,set_model
,get_year
,set_year
). - The attributes
__model
and__year
are private (denoted by double underscores), which means they cannot be accessed directly from outside the class. - The
get_model
,set_model
,get_year
, andset_year
methods are the public interface, allowing controlled access to the private attributes. - for my example with class check my github projects
Encapsulation helps in:
- Maintaining the integrity of the object’s data.
- Making the code more maintainable and flexible.
- Increasing the security of the application.
Advanced Example: The BankAccount Class
For a more comprehensive understanding, consider this examzzple:
class BankAccount:
def __init__(self, account_number, name, balance):
self.__account_number = account_number # private attribute
self.__name = name # private attribute
self.__balance = balance # private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return f"{amount} deposited. New balance: {self.__balance}"
else:
return "Invalid amount"
def withdraw(self, amount):
if 0 < amount <= self.__balance:
self.__balance -= amount
return f"{amount} withdrawn. New balance: {self.__balance}"
else:
return "Insufficient balance or invalid amount"
def get_balance(self):
return f"Account balance: {self.__balance}"
# Creating an account
my_account = BankAccount("12345678", "Deniz Halil", 1000)
# Deposit and withdrawal operations
print(my_account.deposit(500)) # Deposit $500
print(my_account.withdraw(200)) # Withdraw $200
print(my_account.get_balance()) # Check balance
In this example:
- The
BankAccount
class encapsulates data such as the account number, customer name, and balance, along with methods to operate on these data (deposit, withdraw, check balance). - The attributes
__account_number
,__name
,__balance
are private, meaning they cannot be directly accessed from outside the class. - Methods like
deposit
,withdraw
, andget_balance
allow safe operations on the account. For instance, thewithdraw
method prevents withdrawing money if there is an insufficient balance.
This approach enhances the security of the account information and prevents erroneous transactions. Encapsulation in this way maintains data integrity and organization, while also preventing misuse.
Conclusion
Encapsulation is a powerful concept in programming that greatly contributes to the creation of secure, maintainable, and efficient code. By controlling how data is accessed and modified, it lays the groundwork for robust and reliable software applications. Whether in simple or complex systems, encapsulation proves to be an indispensable tool in a programmer’s toolkit.