Introduction:
C++ is a powerful programming language with a wide user base and applications in various fields. Its flexible and robust syntax structure allows programmers to manage complex projects and write more efficient code. In this article, we will learn about the syntax structure in the C++ programming language to understand how the fundamental components work.
Learning Objectives:
- Defining variables and data types in the C++ language.
- Performing arithmetic operations using basic operators.
- Directing program flow with control structures.
- Creating and using functions.
- Managing data structures through arrays and pointers.
- Understanding the fundamentals of object-oriented programming using classes and objects.
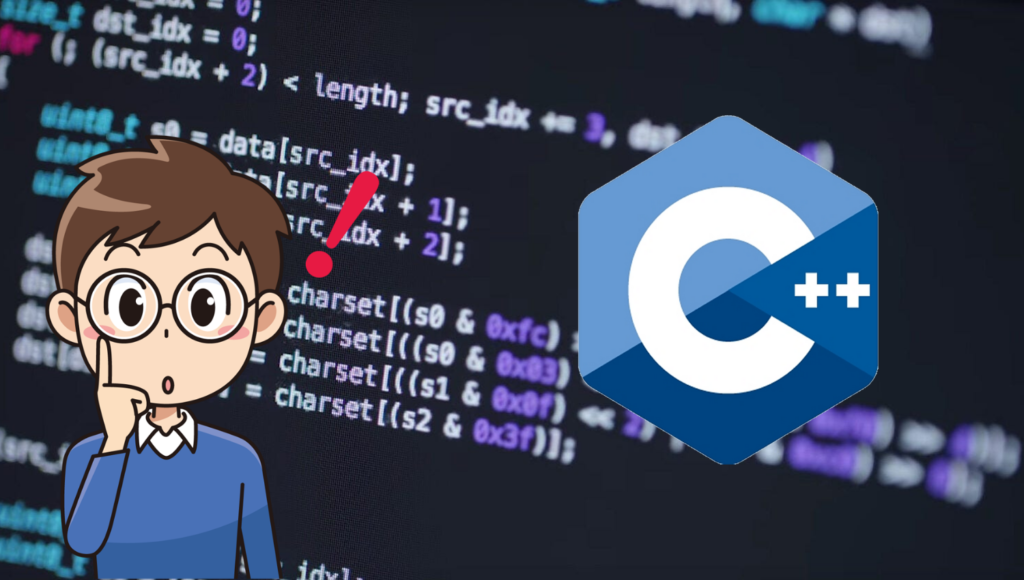
Learning Syntax Structure:
The syntax structure in C++ programming language is broad. Essentially, variables and data types are defined, followed by performing operations between these variables using operators. Control structures are used to direct the flow of the program, while functions modularize the code. Arrays and pointers are used to manage data structures, and classes and objects form the foundation of object-oriented programming. Let’s now explore some examples together and here Beginner Projects in C++ Programming Language.
- Variables and Data Types: Variables are used to store data. For example:
int number = 5;
double pi = 3.14;
char letter = 'a';
- Operators: C++ includes a variety of operators, such as arithmetic operators (+, -, *, /), comparison operators (==, !=, <, >, <=, >=), and logical operators (&&, ||, !).
- Control Structures: Control structures are used to manage program flow. These include if-else statements, switch-case blocks, and loops (for, while, do-while).
if (number > 0) {
// code
} else if (number == 0) {
// code
} else {
// code
}
switch (letter) {
case 'a':
// code
break;
case 'b':
// code
break;
default:
// code
}
for (int i = 0; i < 10; ++i) {
// code
}
while (number > 0) {
// code
}
do {
// code
} while (number > 0);
- Functions: Functions modularize the code.
int add(int x, int y) {
return x + y;
}
- Arrays and Pointers: Arrays store a collection of elements of the same type. Pointers store the memory address of a variable.
int array[5] = {1, 2, 3, 4, 5};
int *ptr = &number;
- Classes and Objects: Classes and objects are used for object-oriented programming.
class Student {
public:
int id;
string name;
void greet() {
cout << "Hello, I'm " << name << "!\n";
}
};
Student student1;
student1.id = 123;
student1.name = "John";
student1.greet();
This summarizes the general syntax structure in the C++ programming language. However, C++ includes many more features beyond these elements.
Conclusion:
Learning the syntax structure in C++ programming language is an important step for programmers. It enables them to work on more complex projects and manage their code more efficiently. The fundamental components discussed in this article form the building blocks of the C++ language and provide a solid foundation for transitioning to more advanced topics. By utilizing this foundational knowledge, you can practice and enhance your skills in C++ programming and develop your own projects.