Introduction
In today’s interconnected world, computer networks form the backbone of data transmission across the globe. Within these networks, vast amounts of data are constantly being exchanged. Understanding network traffic is crucial for various applications, including network security, troubleshooting, and monitoring network performance. Scapy, an open-source Python library, provides a powerful toolset for manipulating network protocols. In this article, we will introduce you to the basics of monitoring network traffic using Scapy and guide you through a simple code example.
What is Scapy?
Scapy is an open-source network protocol manipulation library written in Python. Not only can Scapy be used to generate network traffic, but it also allows you to capture and analyze network packets. This library is a valuable resource for network engineers, cybersecurity professionals, and network analysts.
Network Traffic Monitoring Code
You can use Scapy to monitor network traffic by implementing the following Python code:
from scapy.all import *
interface = 'eth0'
def packet_handler(packet):
if packet.haslayer(IP):
src_ip = packet[IP].src
dst_ip = packet[IP].dst
print(f"IP Packet - Source IP: {src_ip}, Destination IP: {dst_ip}")
sniff(iface=interface, prn=packet_handler)
This code listens on the specified network interface and prints captured IP packets to the screen.
Code Breakdown
from scapy.all import *
: Imports the Scapy library.interface = 'eth0'
: Specifies the network interface to listen on.def packet_handler(packet):
: Defines a function that will be called for each captured packet.if packet.haslayer(IP):
: Processes only IP packets.src_ip = packet[IP].src
anddst_ip = packet[IP].dst
: Extracts the source and destination IP addresses from the packet.print(f"IP Packet - Source IP: {src_ip}, Destination IP: {dst_ip}")
: Displays the packet information on the screen.sniff(iface=interface, prn=packet_handler)
: Listens on the specified network interface and passes packets to thepacket_handler
function.
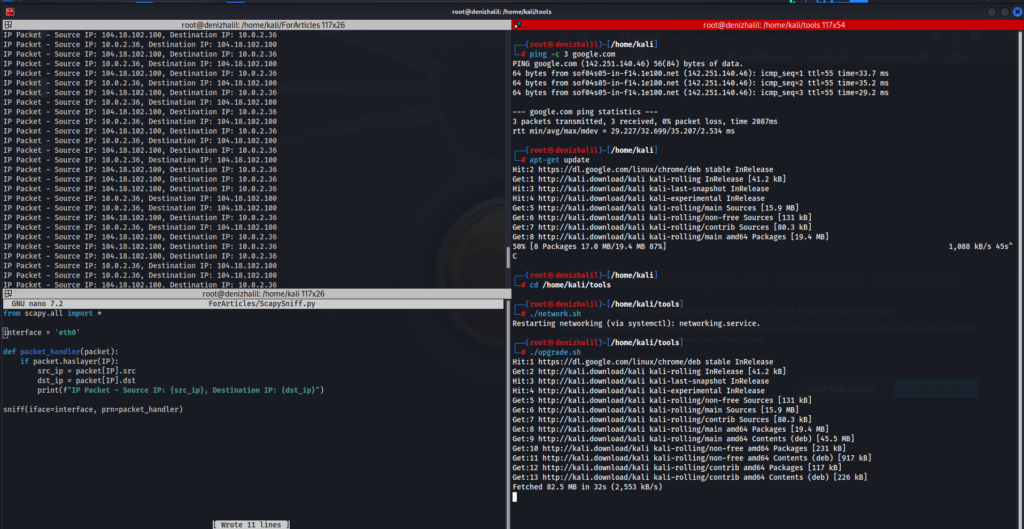
Conclusion
In this article, we introduced the concept of monitoring network traffic using Scapy. With its robust capabilities, Scapy enables you to capture, analyze, and even generate network traffic. This basic code example serves as a starting point for more complex network monitoring and analysis projects. Network traffic monitoring is essential in various scenarios, including network security, troubleshooting, and performance monitoring, and Scapy provides a powerful tool for these tasks. Explore the world of network traffic analysis with Scapy and enhance your network management skills.
For any inquiries or further assistance, please feel free to contact us.
thank you for sharing