Introduction
As computer systems advance, cybersecurity threats become increasingly complex. Among these threats, buffer overflow attacks pose a significant risk to many software and systems. This article will discuss how this attacks occur, the potential dangers they pose, and the preventive measures that can be taken to mitigate such attacks.
- What is Buffer Overflow?
Buffer overflow refers to the situation where a buffer, a region of memory in a software, exceeds its boundaries due to excessive input. This situation can be exploited by an attacker to compromise the target system or execute unintended code. this attacks often result from programming errors and can be leveraged by malicious actors for nefarious purposes.
Let’s consider an example of a buffer overflow attack. Imagine a simple code snippet written in C:
#include <stdio.h>
void vulnerableFunction(char* input) {
char buffer[10];
strcpy(buffer, input);
printf("Received data: %s\n", buffer);
}
int main() {
char userInput[100];
printf("Enter some data: ");
gets(userInput);
vulnerableFunction(userInput);
return 0;
}
In the above example, a function called vulnerableFunction
copies user input into a buffer named buffer
with a size of 10 characters. However, when the input provided by the user exceeds 10 characters, a buffer overflow occurs, leading to a security vulnerability in the target system.
- Dangers of Buffer Overflow Attacks
this attacks can have severe consequences on a target system. Below are some examples of the dangers they can pose:
a. Remote Code Execution: Attackers can achieve remote code execution by injecting malicious code into the target system. This allows attackers to take control of the system and perform unauthorized operations.
b. Exploitation of Vulnerabilities: Buffer overflow can lead to the exploitation of specific vulnerabilities within the target system. For instance, attackers can use such attacks to gain unauthorized access, crash the system, or access sensitive data.
c. Denial-of-Service (DoS): Attackers can overload the target system through the attacks, disrupting the normal functioning of the system and causing a denial-of-service condition.
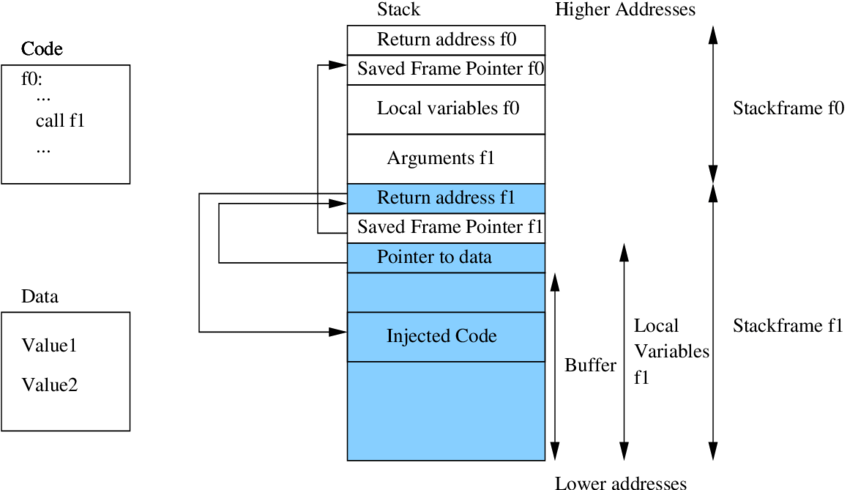
Prevention of Buffer Overflow Attacks
To minimize the impact of buffer overflow attacks and ensure system security, the following measures can be implemented:
a. Software Updates: Software developers should regularly update their software to prevent attacks. These updates help prevent attackers from exploiting known security vulnerabilities.
b. Error Checking and Code Review: Error checking and code review are crucial during the software development process. These steps can be utilized to identify potential errors and security vulnerabilities.
c. Memory Management: Effective memory management is essential. Proper memory allocation and controlling buffer sizes help prevent attacks.
d. Data Validation and Limitation: Input data should be validated and limited using mechanisms such as input sanitization and boundary checks. This prevents attackers from injecting malicious data into the target system.
e. Stack Protection Mechanisms: Stack protection mechanisms employed by compilers and runtime environments aid in detecting and preventing buffer overflow attacks. One example is the Stack-Smashing Protection (StackGuard) technique.
Here is an example code snippet demonstrating buffer overflow prevention:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void safeFunction(char* input) {
char buffer[10];
strncpy(buffer, input, sizeof(buffer)-1);
buffer[sizeof(buffer)-1] = '\0';
printf("Received data: %s\n", buffer);
}
int main() {
char userInput[100];
printf("Enter some data: ");
fgets(userInput, sizeof(userInput), stdin);
safeFunction(userInput);
return 0;
}
In the above example, the strncpy
function is used to safely copy the input data into the buffer
while limiting it to the size of the buffer. Additionally, a null character is added at the end of the buffer to prevent.
Popular Tools for Buffer Overflow Analysis and Defense
Several popular tools are available for performing that attacks analysis and strengthening system defenses. These tools include:
a. Metasploit Framework: Metasploit is a widely used tool for security testing and simulating attacks. It includes various exploit modules and can be used to perform attacks, along with other attack techniques.
b. Immunity Debugger: Immunity Debugger is a tool specifically designed for security testing of Windows-based applications. It is effective for analyzing and debugging attacks.
c. GDB: GDB (GNU Debugger) is a widely used debugging tool for Unix and Linux-based systems. It can be utilized to detect and analyze security vulnerabilities, including issues.
d. IDA Pro: IDA Pro is a professional-grade tool used for software analysis and reverse engineering. It aids in identifying and analyzing and security vulnerabilities.
Conclusion
Buffer overflow attacks pose a significant threat to computer systems and software. These attacks enable attackers to compromise the target system, inject malicious code, or access sensitive data. However, by implementing software updates, error checking, memory management, data validation, and utilizing stack protection mechanisms, that attacks can be mitigated. With the incorporation of these preventive measures and best security practices, the security and integrity of computer systems can be maintained.
Pretty! This has been a really wonderful post. Many thanks for providing these details.
Hi there to all, for the reason that I am genuinely keen of reading this website’s post to be updated on a regular basis. It carries pleasant stuff.