Introduction
In the realm of cybersecurity, password cracking and vulnerability identification play pivotal roles. One of the foundational tools utilized in these processes is wordlists. Wordlists are files containing various combinations and character sets that potential passwords may include. Programming languages like Python offer handy tools for generating wordlists
Learning Objectives
- Learn how to create wordlists using Python.
- Understand the importance of wordlists in cybersecurity.
- Develop a simple wordlist generator program.
The Role of Wordlists in Cybersecurity
Cyber attackers often use wordlists in password cracking attacks. These wordlists typically include common passwords, dictionaries, combinations, and even custom word sets. Password cracking attacks attempt to gain unauthorized access to user accounts using these wordlists. Therefore, cybersecurity experts encourage the use of strong and unique passwords while also implementing security measures to protect their systems against such attacks(John the Ripper Usage Cheat Sheet: A Quick Guide).
Let’s Start Writing Our Code
Let’s develop a simple wordlist generator program using Python. This program will generate all combinations of a specified character set for a given word length.
- Importing Libraries:
import itertools
import argparse
These lines import the necessary libraries for our code. The itertools
module will be used to generate combinations, while the argparse
module will handle command-line arguments.
Programming Symbols Stickers 50Pcs
Waterproof, Removable, Cute, Beautiful, Stylish Teen Stickers, Suitable for Boys and Girls in Water Bottles, Phones, Suitcase Vinyl
$5.59 on Amazon- Defining the WordListGenerator Class:
class WordListGenerator:
def __init__(self, min_length=1, max_length=3, char_set='abc'):
self.min_length = min_length
self.max_length = max_length
self.char_set = char_set
def generate_word_list(self):
word_list = []
for length in range(self.min_length, self.max_length + 1):
for combination in itertools.product(self.char_set, repeat=length):
word = ''.join(combination)
print(word)
word_list.append(word)
return word_list
In this section, we define a class called WordListGenerator
. This class will be used to generate all combinations of a specified character set for a given word length. The __init__
method is the constructor of the class, which sets default minimum and maximum lengths and character sets. The generate_word_list
method generates all combinations and prints them to the screen(Password Security with Machine Learning).
- Defining the main Function:
def main():
parser = argparse.ArgumentParser(description="wordlist generator by DenizHalil")
parser.add_argument("--min", dest="min", type=int, required=True, help="Enter minimum word length")
parser.add_argument("--max", dest="max", type=int, required=True, help="Enter maximum word length")
parser.add_argument("--charset", dest="char_set", default="abc", help="Character set (default: 'abc')")
return parser.parse_args()
In this section, we define a function named main
. This function processes command-line arguments and prompts the user to enter the minimum word length, maximum word length, and character set.
- Running the Main Code:
if __name__ == "__main__":
args = main()
generator = WordListGenerator(args.min, args.max, args.char_set)
word_list = generator.generate_word_list()
In this section, we call the main
function, create an instance of the WordListGenerator
class with the arguments obtained from the user, and generate the wordlist using the generate_word_list
method.
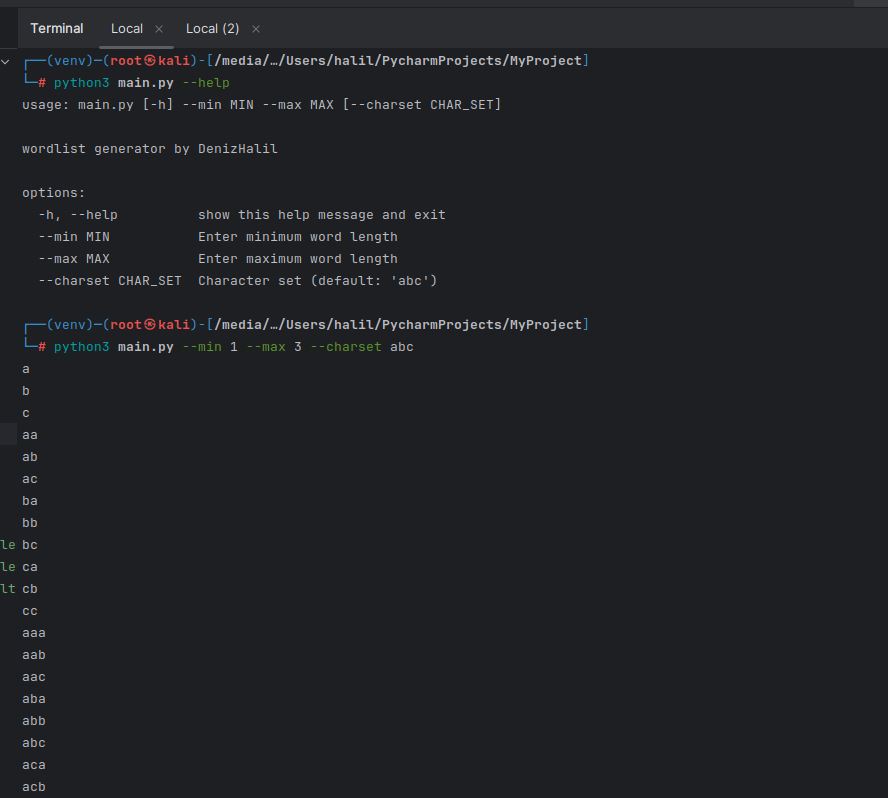
Conclusion
In this article, we provided a general introduction to creating wordlists using Python. We understood the importance of wordlists in cybersecurity and developed a simple wordlist generator program. Such tools can support cybersecurity experts in their efforts to strengthen password security while also helping to prevent potential attacks by cyber adversaries. Therefore, the use and development of such tools are crucial for anyone working in the field of cybersecurity.